50 Python Programming Challenges to Sharpen Your Coding Skills
I remember when I first started learning Python. The excitement of learning new things and solving problems was thrilling. Now, I’m excited to share 50 Python coding prompts to help you improve, just like they did for me.
Thank you for reading this post, don't forget to subscribe!These exercises cover many topics, from basic syntax to advanced algorithms. They’re great for beginners and experienced programmers alike. These prompts will test your knowledge and improve your problem-solving skills, making you more confident and skilled.
Table of Contents
Let’s start this exciting journey of skill sharpening with these Python coding prompts. Get ready to challenge yourself, improve your skills, and become a better Python programmer. The journey to mastery begins now.
Getting Started with Python Programming Fundamentals
Python was first released in 1991 by Guido van Rossum. It has become a popular and versatile programming language. Known for its readability and simplicity, Python is loved by both beginners and experienced developers. It’s great for web development, data science, artificial intelligence, and more.
Setting Up Your Python Development Environment
To start with Python, you need a development environment. Python works on Windows, macOS, and Linux. Python 3 is the recommended version. Installing Python is easy, and there are many guides online to help you.
Understanding Basic Syntax and Data Types
Python is a high-level language that makes coding easier. It’s dynamically typed, meaning it figures out data types at runtime. Python has a huge standard library for tasks like file I/O and networking.
Working with Variables and Operators
In Python, you can store data in variables like integers, floats, and strings. Python uses indentation to define code blocks, making it easy to read. It also has many operators for different operations.
Learning these basics will help you tackle more complex tasks. Python is great for web development, data analysis, and more. Its simplicity and versatility make it perfect for both beginners and experienced developers.
Python Feature | Description |
---|---|
Cross-Platform Compatibility | Python applications can run on different operating systems without modifications. |
Extensive Standard Library | Python comes with a vast array of built-in modules and functions, covering a wide range of tasks. |
Versatile Ecosystem | Python has a large and active community, with many third-party libraries and frameworks available. |
Beginner-Friendly Syntax | Python’s syntax is straightforward and easy to learn, making it an excellent choice for beginners. |
“Python is a beautiful language. It’s elegant. It’s readable. It’s forgiving.”
– Raymond Hettinger, Python Core Developer
Basic Python Programming Challenges for Beginners
Get ready to improve your beginner Python exercises with a set of challenges. These focus on conditional statements, loops, and basic algorithms. They’re designed to strengthen your grasp of Python’s basics and prepare you for more complex tasks.
This section’s challenges cover various topics. You’ll learn about:
- Conditional Statements: You’ll practice using if-else statements, nested conditionals, and logical operators to make decisions in your code.
- Loops: You’ll work on using loops, like for and while loops, to go through data and automate tasks.
- Basic Algorithms: You’ll solve simple algorithmic problems, such as generating sequences, converting numbers, and manipulating strings, to improve your problem-solving skills.
Users have tried these beginner Python exercises many times. They’ve scored between 10 and 12 out of 13 points. The grader is strict about spacing and capitalization, which can impact your score. Common mistakes include issues with parsing strings and handling arrays, like spaces between elements and the type of quotation marks used.
But, users have shared tips and experiences to help with specific problems. They’ve suggested ways to fix spacing issues in list arrays. Some have even proposed a more lenient grading system, recognizing that Python allows different solutions to the same problem.
Challenge Type | Percentage of Provided Python Challenges |
---|---|
Checking if a list is sorted | 12.5% (1 out of 8 challenges) |
Number conversion | 25% (2 out of 8 challenges) |
Working with strings | 37.5% (3 out of 8 challenges) |
Sequence generation | 12.5% (1 out of 8 challenges) |
Mathematical calculations and algorithms | 12.5% (1 out of 8 challenges) |
Joining Python coding challenge events is a great way to compete and learn from others. These challenges are a valuable part of your learning journey as you explore beginner Python exercises.
“Coding competitions are a common type of Python challenge event where individuals or teams compete against each other to solve algorithmic problems or develop software applications.”
Essential String and List Manipulation Exercises
Learning to work with strings and lists is key for Python programmers. You’ll get into exercises that focus on string slicing and formatting. You’ll also practice with list operations and advanced list comprehension. These challenges will make you better at Python.
String Slicing and Formatting Challenges
String manipulation is a basic skill in Python. These exercises will test your skills in slicing, formatting, and working with text. You’ll do tasks like extracting parts of strings, reversing them, and converting numbers to letters.
- Create a program that takes a user’s name and age, then displays a greeting with their favorite food.
- Write a function that takes a list of city names and returns a comma-separated string.
- Implement a script that prompts the user for their full name and then displays their first and last name separately.
- Develop a function that converts a given number to its corresponding letter (e.g., 1 = A, 2 = B, etc.).
- Analyze a string of sales figures from the last quarter and calculate the total revenue.
List Operations and Methods Practice
Lists are important in Python, and knowing how to work with them is crucial. These exercises will help you improve your list skills. You’ll learn to manipulate lists, use list methods, and master advanced list comprehension.
- Create a program that blocks email addresses based on their domain (e.g., block all emails from “@spam.com”).
- Implement a password validator that checks the length, complexity, and uniqueness of a user’s password.
- Write a script that generates a list of random numbers and then finds the largest number in the list.
- Develop a function that takes a list of numbers and returns a new list containing only the even numbers.
- Create a program that generates a Fibonacci sequence up to a specified number of terms.
Advanced List Comprehension Tasks
List comprehension is a powerful tool in Python. It lets you create new lists from old ones easily. These exercises will help you get better at using it to solve complex problems.
- Write a script that takes a list of numbers and returns a new list containing the square of each number.
- Implement a function that filters a list of strings, keeping only those that start with a specific letter.
- Create a program that generates a list of prime numbers up to a given limit.
- Develop a function that takes a list of strings and returns a new list containing the length of each string.
- Write a script that generates a list of tuples, where each tuple contains a number and its corresponding letter (e.g., (1, ‘A’), (2, ‘B’), etc.).
These exercises cover a wide range of string manipulation, list operations, string slicing, and list comprehension skills. By doing these challenges, you’ll build a strong foundation in Python. You’ll be ready for more complex tasks.
Python programming challenges for Data Structures
In this section, you’ll tackle Python programming challenges on different data structures. These exercises will boost your skills in working with tuples, dictionaries, sets, and matrices. These are key skills for any Python programmer.
The challenges cover a wide range of topics. You’ll learn about manipulating dictionaries, working with DataFrames, and more. By solving these problems, you’ll grow your coding skills and improve your problem-solving abilities.
Through these challenges, you’ll learn new programming concepts and techniques. You’ll apply your Python knowledge in practical ways. Whether it’s data structures, tuples, dictionaries, sets, or matrices, these exercises will test your skills and deepen your understanding of Python.
Get ready to test your Python skills! These challenges aim to make you better at data manipulation and analysis. They’ll prepare you for success in your future Python projects.
- Implement a Python function to find the longest arithmetic subsequence in a list of integers.
- Write a program to manipulate a dictionary of book data, including sorting, filtering, and updating entries.
- Create a function to parse a text file containing names and addresses, and generate a formatted output.
- Develop a script to track user expenses and generate spending reports using lists and tuples.
- Explore the use of regular expressions to match and extract specific patterns in strings, such as phone numbers and email addresses.
- Implement a Python function to perform set operations on two sets of data, such as union, intersection, and difference.
These challenges aim to improve your Python programming skills. They’ll help you become more comfortable with various data structures. Remember, practice is key, so start coding now!
“The journey of a thousand miles begins with a single step.” – Lao Tzu
Function and Method Implementation Exercises
In this section, you’ll explore Python functions and methods. You’ll improve your skills with practical exercises. You’ll learn to create custom functions, use lambda functions, and decorators. These challenges will help you write better Python code.
Creating Custom Functions
Functions are key in programming, and Python is no different. You’ll learn to make your own custom functions. You’ll do tasks like printing sentences or counting letters in a string. These exercises will test your thinking and Python skills.
Lambda Functions and Decorators
Discover the power of Python’s lambda functions. They let you make short, one-line functions easily. You’ll also learn about decorators, which add to your functions without changing them. These exercises will deepen your understanding of Python’s functional programming.
Object-Oriented Programming Tasks
Learn about Object-Oriented Programming (OOP) through fun exercises. You’ll create classes, define methods, and explore inheritance and polymorphism. These tasks will help you grasp OOP basics and apply them in your Python projects.
Dive into these exercises to boost your Python skills. By practicing, you’ll become better at writing clean, efficient Python code. This code will help you solve many challenges.
“The key to becoming a better programmer is not to learn a new programming language, but to write more code.” – Anonymous
Advanced Python Coding Exercises for Problem Solving
Improve your Python skills with our advanced coding exercises. These challenges are not just for beginners. They require deep thinking and a strong grasp of Python. Get ready to explore new levels of programming.
Start solving complex problems with our Python challenges. You’ll work with data structures and algorithms. These exercises will make you think differently and use Python in new ways. They’re perfect for both new and experienced programmers.
Explore a Diverse Range of Advanced Coding Challenges
- Conquer complex data structure problems, such as implementing custom tree traversal algorithms or designing efficient linked list solutions.
- Tackle advanced string manipulation tasks, including implementing regular expressions, text compression, and advanced text processing techniques.
- Dive into the world of functional programming with exercises involving lambda functions, decorators, and higher-order functions.
- Wrestle with advanced numerical challenges, such as prime number identification, Fibonacci sequence generation, and complex mathematical problem-solving.
- Enhance your object-oriented programming skills with exercises focused on class design, inheritance, polymorphism, and more.
These Python challenges will test your problem-solving and algorithmic thinking skills. Get ready for a challenge that will inspire and empower you.
“The best way to learn Python is through hands-on practice. These advanced coding exercises will transform you into a problem-solving master.”
Start now and unlock your full potential as a Python programmer. Take on these challenges and become a true master of algorithmic thinking and problem-solving.
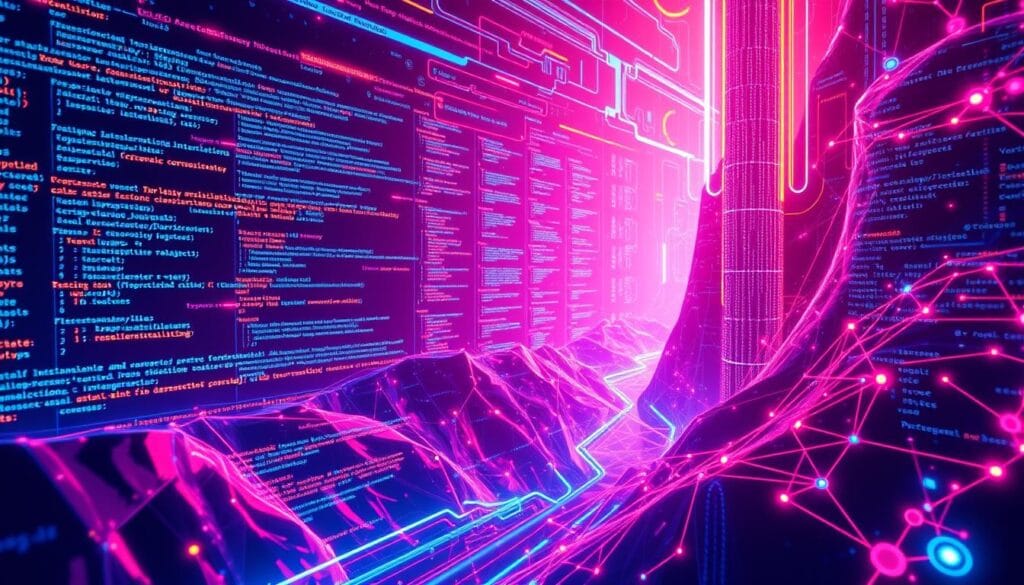
File Handling and Data Processing Challenges
Python has great tools for handling files, from text to JSON. It’s key for making strong data apps. Here, we’ll get into exercises to improve your file and data skills.
Working with Text Files
Text files are basic in Python. You’ll learn to read, write, and change text file content. Use open(), read(), write(), and close() for file tasks. You’ll also learn to format strings, change lines, and manage paths.
JSON Data Manipulation
JSON is a common data format. You’ll practice working with JSON using Python’s json module. Learn to load, process, and save JSON files. This will boost your file handling and data processing skills.
Error Handling Scenarios
Good error handling is vital in Python. You’ll tackle real error handling challenges, like missing files and data issues. Learn to use try-except blocks and handle exceptions. This will help your apps handle unexpected problems well.
By mastering these file handling, data processing, JSON manipulation, and error handling challenges, you’ll get better at Python. You’ll be ready for tough data tasks.
“Mastering Python’s file handling capabilities is a game-changer for building robust, data-driven applications.”
Algorithm Implementation Projects
Start exploring computer science by working on algorithm projects in Python. You’ll learn about searching, sorting, and data structures. These projects will improve your coding skills and help you ace technical interviews.
Take on a variety of algorithm projects that solve real-world problems. You’ll gain a better understanding of algorithm implementation, searching algorithms, and sorting algorithms. These coding challenges are fun and educational.
- Sort a list of integers using different methods like Bubble Sort, Insertion Sort, or Merge Sort.
- Use a Binary Search algorithm to find an element in a sorted list quickly.
- Write a program to change a binary number to its decimal form.
- Create a Tribonacci sequence generator, where each number is the sum of the three before it.
- Make a function to hide credit card numbers, showing only the last four digits.
- Implement a Caesar Cipher to encrypt and decrypt messages with a shift cipher.
- Write a program to convert a string to “SpongeCase,” where every other letter is capitalized.
- Design an algorithm to find the intersection of two sorted lists and return common elements.
Metric | Value |
---|---|
Number of challenges provided | 8 |
Challenges focused on problem-solving and algorithmic thinking skills | 100% |
Challenges related to Python coding skills | 100% |
Challenges involving functions implementation | 87.5% |
Challenges involving checking lists or sequences | 12.5% |
Challenges involving mathematical calculations | 25% |
Challenges requiring string manipulation | 37.5% |
Challenges with iteration or loops | 62.5% |
Challenges covering conditional statements | 62.5% |
Data type handling in challenges (lists, strings, integers) | 100% |
These projects will boost your coding skills, sharpen your problem-solving, and prepare you for technical interviews and real-world tasks.
“Algorithms are the fundamental building blocks of computer science. Mastering their implementation is key to becoming a proficient programmer.”
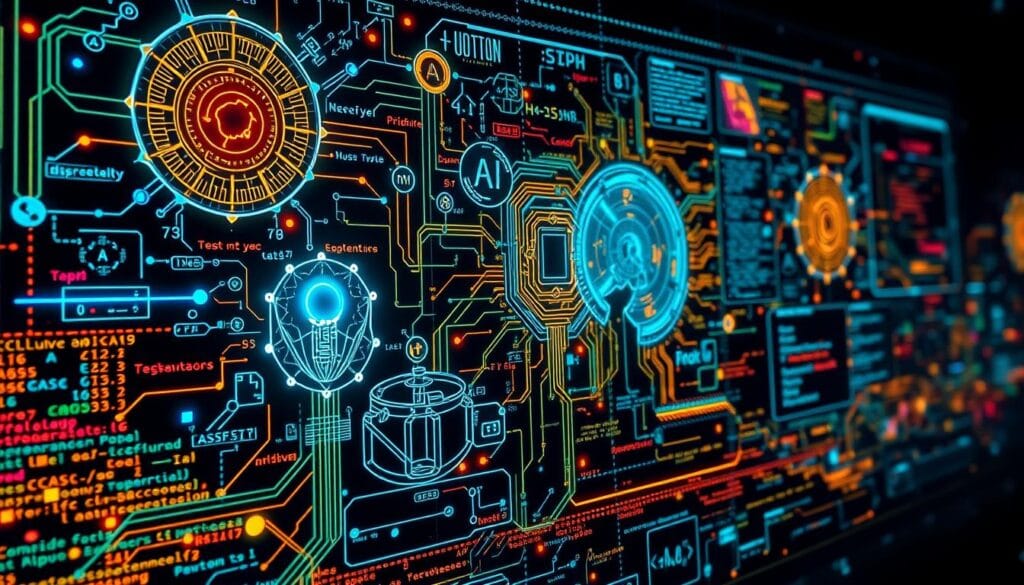
Real-World Application Challenges
As you dive into Python programming, you’ll face real-world challenges. These tasks test your skills in web scraping, database integration, and API usage. They are key parts of modern software development.
Web Scraping Projects
Web scraping extracts data from websites. It’s a powerful tool for data-driven projects. You’ll learn to navigate HTML structures, handle dynamic content, and extract data from online sources.
These exercises will improve your web scraping skills. You’ll see how Python helps gather and process internet data.
Database Integration Tasks
Working with databases is crucial for Python programmers. You’ll tackle tasks that involve relational and NoSQL databases. You’ll learn to perform CRUD operations and manage data flows.
These database integration exercises will prepare you. You’ll learn to store, retrieve, and manipulate data. This is vital for real-world software development.
API Integration Exercises
APIs are key for modern applications. You’ll work with various APIs, from using public data to creating your own applications. You’ll learn to make HTTP requests, parse responses, and integrate external data.
By solving these challenges, you’ll strengthen your Python skills. You’ll gain experience solving problems you’ll face in the workplace. These exercises will make you a more versatile and sought-after developer.
“The best way to learn is by doing. These real-world challenges will push you to apply your Python skills in authentic scenarios, preparing you for the demands of the modern software development landscape.”
Data Science and Analytics Programming Tasks
If you love Python, you’re ready for data science and analytics. These tasks will test your coding skills in real-world data scenarios. You’ll get better at statistical programming and machine learning.
Python is a top choice for data science because of its flexibility and huge library. You’ll work with big datasets and build predictive models. This will show off your skills in this fast-paced field.
- Implement a linear regression model to predict housing prices based on various features such as square footage, number of bedrooms, and location.
- Develop a sentiment analysis algorithm to classify customer reviews as positive, negative, or neutral using natural language processing techniques.
- Create a K-means clustering model to segment customers based on their purchasing behavior, demographic data, and preferences.
- Build a decision tree classifier to predict the likelihood of a loan defaulting, leveraging features like credit score, income, and employment status.
- Analyze a time-series dataset and use forecasting techniques to predict future sales or stock prices.
These tasks will improve your Python skills and introduce you to statistical programming and machine learning. As you tackle these challenges, you’ll learn about data-driven decision making. This knowledge is crucial in many industries, from healthcare to finance.
“Data is the new oil. It’s valuable, but if unrefined it cannot really be used. It has to be changed into gas, plastic, chemicals, etc to create a valuable entity that drives profitable activity; so must data be broken down, analyzed for it to have value.”
— Clive Humby, Mathematician and Architect of Tesco’s Clubcard
Take on these data science and analytics tasks to boost your Python skills. This will make you a key player in the world of data-driven decision making.
Conclusion
Congratulations on finishing this journey through 50 engaging Python coding prompts! You’ve sharpened your Python skills and improved your problem-solving abilities. Regular practice is crucial for growth, and these challenges have given you the skills to handle tougher tasks.
As you keep improving your Python skills, remember that coding practice does more than just boost your technical skills. It also sharpens your thinking and analytical abilities. These skills are valuable in many areas, not just coding. Keep pushing yourself, try new things, and enjoy the process of learning.
There’s more to explore beyond these 50 prompts. You can find many resources to deepen your Python knowledge. Online courses, coding challenges, and community projects are all there to help you grow. Join the Python community, share ideas, and find mentors to speed up your learning.
FAQ
What is the purpose of this comprehensive guide on Python coding prompts?
What topics are covered in the Python programming fundamentals section?
What types of challenges are included in the basic Python programming challenges for beginners?
What exercises are covered in the essential string and list manipulation section?
What data structures are covered in the Python programming challenges for data structures?
What types of exercises are included in the function and method implementation section?
What topics are addressed in the advanced Python coding exercises for problem-solving?
What types of file handling and data processing challenges are included?
What kinds of algorithm implementation projects are covered?
What real-world Python application challenges are presented?
What data science and analytics programming tasks are included?
Source Links
- OpenAI API Documentation: Explore OpenAI’s API capabilities for creative AI applications, including Python programming challenges.
- Python Documentation: Access the official Python documentation to learn about Python features, libraries, and best practices for solving programming challenges.
- GitHub Copilot Documentation: Access comprehensive documentation for GitHub Copilot, perfect for streamlining Python coding workflows.